11. ๐จ Bonus (editing annotations)
Let's first get all the categories (i.e., labels) in our dataset
dataset.categories
""" Output:
[Category(name='animal', color='#1F77B4', id=0),
Category(name='human.pedestrian.adult', color='#FF7F0E', id=1),
Category(name='human.pedestrian.child', color='#2CA02C', id=2),
Category(name='human.pedestrian.construction_worker', color='#D62728', id=3),
Category(name='human.pedestrian.personal_mobility', color='#9467BD', id=4),
Category(name='human.pedestrian.police_officer', color='#8C564B', id=5),
Category(name='human.pedestrian.stroller', color='#E377C2', id=6),
Category(name='human.pedestrian.wheelchair', color='#818181', id=7),
Category(name='movable_object.barrier', color='#BCBD22', id=8),
Category(name='movable_object.debris', color='#17BECF', id=9),
Category(name='movable_object.pushable_pullable', color='#DB0C75', id=10),
Category(name='movable_object.trafficcone', color='#FDB8FD', id=11),
Category(name='static_object.bicycle_rack', color='#614F61', id=12),
Category(name='vehicle.bicycle', color='#310C45', id=13),
Category(name='vehicle.bus.bendy', color='#9B53E7', id=14),
Category(name='vehicle.bus.rigid', color='#666BB0', id=15),
Category(name='vehicle.car', color='#6455B8', id=16),
Category(name='vehicle.construction', color='#1DEFA4', id=17),
Category(name='vehicle.emergency.ambulance', color='#F852C5', id=18),
Category(name='vehicle.emergency.police', color='#24EBB9', id=19),
Category(name='vehicle.motorcycle', color='#3A2C16', id=20),
Category(name='vehicle.trailer', color='#F5EF1B', id=21),
Category(name='vehicle.truck', color='#A32155', id=22)]
"""
We'll edit the annotations of one of the images tagged with weather
:rain
search_result_rain_tag = dataset.search_images(
filter="image_tag:[weather_rain]"
)
display_images(\[search_result_rain_tag[0]], n_images_to_show=1, draw_bboxes=True)
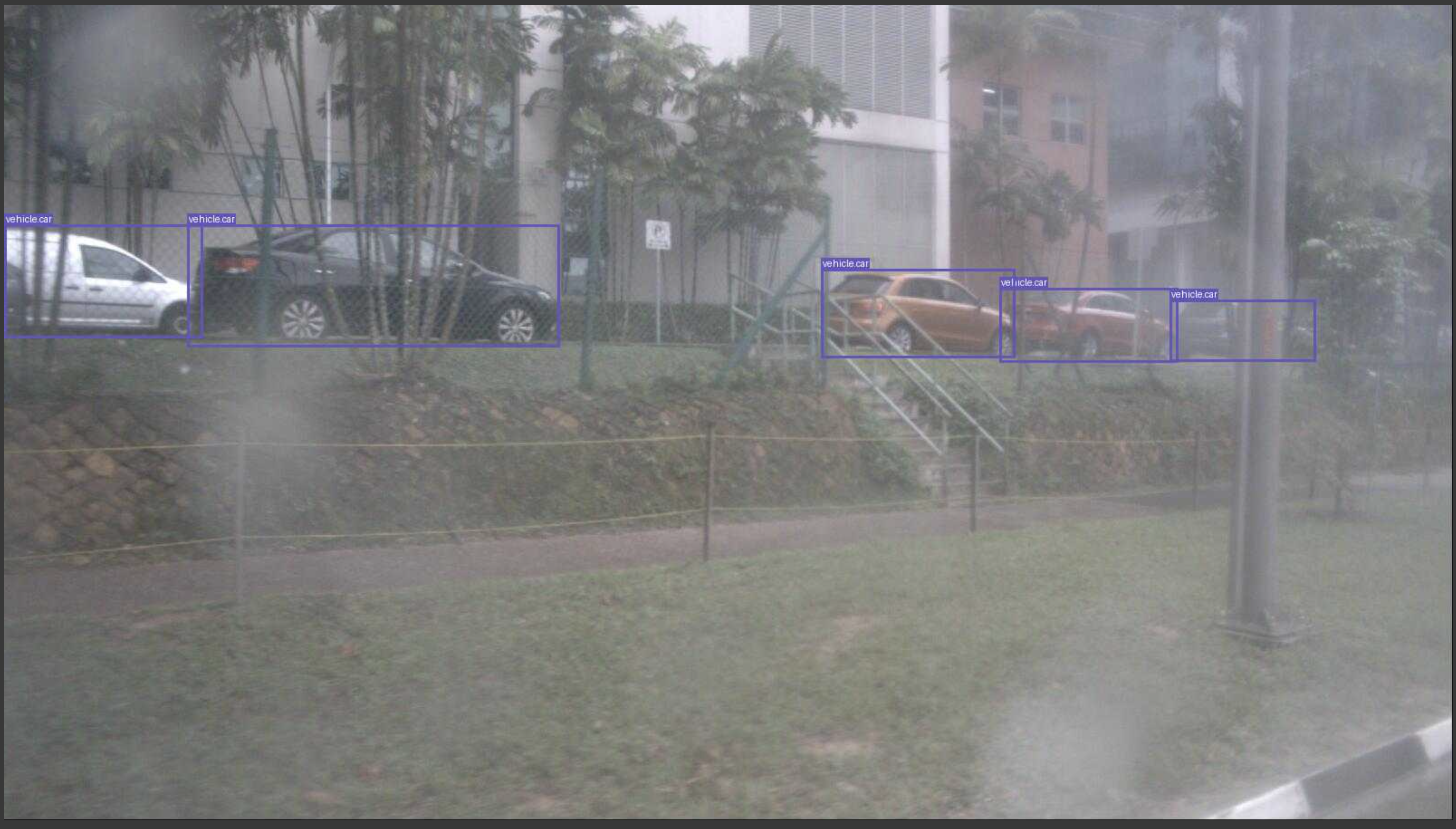
We can get the image key of our image with the next command
search_result_rain_tag[0].key
""" Output:
n003-2018-01-02-14-36-45-0800__CAM_FRONT_LEFT__1514875528239124_jpg.rf.5a38f43dc6e9e03e4ffc697fb9c480de
"""
We can also get the specific coordinates of its bounding box
search_result_rain_tag[0].annotations[0]
From the categories we retrieved before, we specifiy that our annotation belongs to the car
category
car = Category(name="vehicle.car")
car
Next, we define our current and new annotation's coordinates
updated_annotations = [
Annotation(
coordinates=[1099.0, 312.0, 197.0, 82.0],
category=car,
),
Annotation(coordinates=[1199.0, 412.0, 297.0, 182.0], category=car),
]
Finally, we update our image with the new annotation
dataset.update_image(search_result_rain_tag[0].key, updated_annotations)
You can learn more about how to update annotations and images here.
Updated 3 months ago